Face tracking
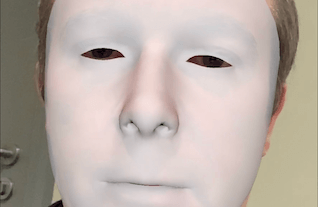
Description
Here we look at ARKits build in ability to track faces.
Notice at the start instead of using a ARWorldTrackingConfiguration
we are using an instance of ARFaceTrackingConfiguration
. It would appear that among other things this sets the initial state of the app to use the front camera.
Video
Code
using ARKit;
using SceneKit;
using System;
using UIKit;
namespace XamarinArkitSample
{
public partial class ViewController : UIViewController
{
private readonly ARSCNView sceneView;
public ViewController(IntPtr handle) : base(handle)
{
this.sceneView = new ARSCNView
{
AutoenablesDefaultLighting = true,
Delegate = new SceneViewDelegate()
};
this.View.AddSubview(this.sceneView);
}
public override void ViewDidLoad()
{
base.ViewDidLoad();
this.sceneView.Frame = this.View.Frame;
}
public override void ViewDidAppear(bool animated)
{
base.ViewDidAppear(animated);
var faceTrackingConfiguration = new ARFaceTrackingConfiguration()
{
LightEstimationEnabled = true,
MaximumNumberOfTrackedFaces = 1
};
this.sceneView.Session.Run(faceTrackingConfiguration);
}
public override void ViewDidDisappear(bool animated)
{
base.ViewDidDisappear(animated);
this.sceneView.Session.Pause();
}
public override void DidReceiveMemoryWarning()
{
base.DidReceiveMemoryWarning();
}
}
public class SceneViewDelegate : ARSCNViewDelegate
{
public override void DidAddNode(ISCNSceneRenderer renderer, SCNNode node, ARAnchor anchor)
{
if (anchor is ARFaceAnchor faceAnchor)
{
var faceGeometry = ARSCNFaceGeometry.Create(renderer.GetDevice());
node.Geometry = faceGeometry;
node.Opacity = 0.8f;
}
}
public override void DidRemoveNode(ISCNSceneRenderer renderer, SCNNode node, ARAnchor anchor)
{
if (anchor is ARFaceAnchor)
{
}
}
public override void DidUpdateNode(ISCNSceneRenderer renderer, SCNNode node, ARAnchor anchor)
{
if (anchor is ARFaceAnchor)
{
var faceAnchor = anchor as ARFaceAnchor;
var faceGeometry = node.Geometry as ARSCNFaceGeometry;
faceGeometry.Update(faceAnchor.Geometry);
}
}
}
}
Next Step : Facial expression detection
After you have mastered this you should try Facial expression detection