Coaching overlay
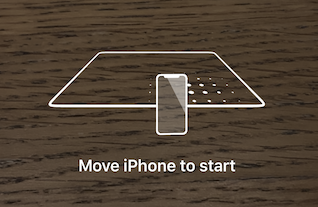
Description
We can use a coaching overlay to prompt the user to move their device around until a goal is met (for example a horizontal plane is found in the scene).
Video
No video yet
Code
using ARKit;
using System;
using UIKit;
namespace XamarinArkitSample
{
public partial class ViewController : UIViewController, IARCoachingOverlayViewDelegate
{
private readonly ARSCNView sceneView;
ARCoachingOverlayView coachingOverlay;
public ViewController(IntPtr handle) : base(handle)
{
this.sceneView = new ARSCNView();
this.View.AddSubview(this.sceneView);
}
public override void ViewDidLoad()
{
base.ViewDidLoad();
this.sceneView.Frame = this.View.Frame;
}
public override void ViewDidAppear(bool animated)
{
base.ViewDidAppear(animated);
this.sceneView.Session.Run(new ARWorldTrackingConfiguration {
PlaneDetection = ARPlaneDetection.Horizontal,
});
coachingOverlay = new ARCoachingOverlayView();
coachingOverlay.Session = sceneView.Session;
coachingOverlay.Delegate = this;
coachingOverlay.ActivatesAutomatically = true;
coachingOverlay.Goal = ARCoachingGoal.HorizontalPlane;
coachingOverlay.TranslatesAutoresizingMaskIntoConstraints = false;
sceneView.AddSubview(coachingOverlay);
// Keeps the coaching overlay in the center of the screen
var layoutConstraints = new NSLayoutConstraint[]
{
coachingOverlay.CenterXAnchor.ConstraintEqualTo(View.CenterXAnchor),
coachingOverlay.CenterYAnchor.ConstraintEqualTo(View.CenterYAnchor),
coachingOverlay.WidthAnchor.ConstraintEqualTo(View.WidthAnchor),
coachingOverlay.HeightAnchor.ConstraintEqualTo(View.HeightAnchor),
};
NSLayoutConstraint.ActivateConstraints(layoutConstraints);
}
public override void ViewDidDisappear(bool animated)
{
base.ViewDidDisappear(animated);
this.sceneView.Session.Pause();
}
public override void DidReceiveMemoryWarning()
{
base.DidReceiveMemoryWarning();
}
}
}
Next Step : Body tracking
After you have mastered this you should try Body tracking